How to Render HTML File in Node.js
Question: How can I render an HTML file using Node.js?
Answer: Rendering an HTML file using Node.js is an essential skill for anyone involved in web development. Node.js, with its fast, scalable, and efficient architecture, allows developers to create web servers and serve static web pages quickly and easily. This guide will walk you through the process step-by-step, covering everything from setting up Node.js to creating a server and serving multiple HTML files.
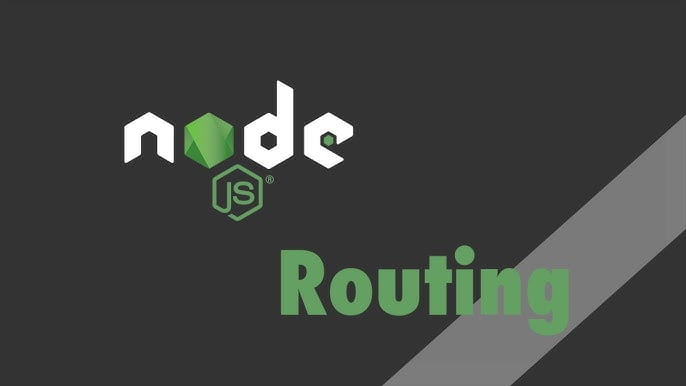
Introduction to Node.js and Its Importance
Node.js is a powerful runtime environment that allows developers to execute JavaScript on the server side. It has become one of the most popular tools for building web applications due to its asynchronous, non-blocking, and event-driven nature. Node.js is widely used by companies like Netflix, Uber, and LinkedIn, making it an important skill to learn.
When building a web application, rendering HTML files is one of the fundamental tasks you’ll need to perform. Whether you’re creating a personal blog, an online store, or a social media platform, you’ll need to serve HTML pages to users. This tutorial will help you understand how to do that using Node.js, and we’ll also discuss some advanced topics like serving multiple HTML files and integrating CSS and JavaScript.
Setting Up Node.js on Your Machine
Before you can start rendering HTML files, you need to have Node.js installed on your computer. Node.js is available for Windows, macOS, and Linux. Follow these steps to set up Node.js:
1. Download and Install Node.js: Visit the official Node.js website and download the installer for your operating system. The website will automatically recommend the LTS (Long-Term Support) version, which is the most stable version for most users.
2. Verify the Installation: After installing Node.js, open your terminal (or command prompt) and type the following command to verify the installation:
node -v
This command will display the version of Node.js installed on your machine. If it shows a version number, you’ve successfully installed Node.js.
3. Install a Code Editor (Optional but Recommended): For writing and managing your code, it’s recommended to use a code editor like Visual Studio Code. It offers features like syntax highlighting, code suggestions, and integrated terminal support, making it easier to work with Node.js projects.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Creating Your First Node.js Server
Now that you’ve set up Node.js, let’s create a simple Node.js server that will render an HTML file. We’ll start by setting up a basic project and writing some code to serve an HTML page.
1. Create a Project Directory: Open your terminal and create a new directory for your project:
mkdir my-node-website
cd my-node-website
This will create a folder named my-node-website
where all your project files will be stored.
2. Initialize Your Node.js Project: Inside the project directory, initialize your Node.js project by running the following command:
npm init -y
This command generates a package.json
file, which manages your project’s dependencies and metadata. The -y
flag automatically accepts the default configuration.
3. Install Express.js (Optional but Highly Recommended): While you can create a server using Node.js’s built-in modules, Express.js simplifies the process. Express is a minimal web framework that provides robust features for building web applications.
To install Express.js, run:
npm install express
With Express.js, you’ll have a more streamlined experience creating and managing routes, handling requests, and rendering HTML files.
Writing the Server Code to Render HTML
Let’s write the server code that will render an HTML file. We’ll start with a basic example and then move on to more advanced scenarios.
1. Create an HTML File: First, create an HTML file named index.html
in your project directory. This file will be served to users when they visit your site:
Welcome to My Node.js Website
Hello, World!
This is an HTML file served by Node.js using Express.
2. Create the Server File: Next, create a file named server.js
in the same directory. This file will contain the code to create a server and serve the index.html
file:
const express = require('express');
const path = require('path');
const app = express();
// Route to serve the HTML file
app.get('/', (req, res) => {
res.sendFile(path.join(__dirname, 'index.html'));
});
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Run the Server: To run your server, open the terminal and execute the following command:
3. Run the Server: To run your server, open the terminal and execute the following command:
node server.js
If everything is set up correctly, your server will start, and you’ll see a message like Server is running on http://localhost:3000
. Open your web browser and navigate to http://localhost:3000
to view your HTML file rendered on the screen.
Understanding the Code
Let’s break down what each part of the server.js
file does:
Importing Express.js and Path Modules:
const express = require('express');
const path = require('path');
These lines import the necessary modules. express
is the framework used to build the web server, and path
is a core Node.js module used to handle and transform file paths.
Creating an Express Application:
const app = express();
This line initializes an Express application. The app
object represents your web application and will be used to define routes and middleware.
Defining a Route:
app.get('/', (req, res) => {
res.sendFile(path.join(__dirname, 'index.html'));
});
This line defines a route for the root URL (/
). When a user visits the root URL, the server responds by sending the index.html
file located in the project directory. The __dirname
variable is a global variable in Node.js that refers to the directory where the script resides.
Starting the Server:
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
This block of code starts the server and listens on the specified port (3000 by default). The process.env.PORT
part allows the server to run on any port specified in the environment variables, which is useful for deployment.
Serving Multiple HTML Files
In many cases, your website will consist of multiple pages. Node.js and Express make it easy to serve multiple HTML files. Here’s how you can do it:
1. Create Additional HTML Files: Let’s say you want to create an about.html
page. Create the file in your project directory:
About Us
About Us
Welcome to the about page of our Node.js website.
2. Define a Route for the New HTML File: Add a new route to your server.js
file to serve the about.html
page:
app.get('/about', (req, res) => {
res.sendFile(path.join(__dirname, 'about.html'));
});
3. Run the Server: Restart your server by running node server.js
again. Now, you can access the about page by navigating to http://localhost:3000/about
.
This ability to serve multiple HTML files allows you to create a full-fledged website with different sections, such as Home, About, Contact, Services, and more.
About Us
About Us
Welcome to the about page of our Node.js website.
Adding CSS and JavaScript to Your Node.js Website
HTML files often need to be styled with CSS and made interactive with JavaScript. Let’s discuss how you can serve static files like CSS and JavaScript using Node.js.
1. Create a Public Directory: In your project directory, create a folder named public
. This folder will contain your static files like CSS, JavaScript, and images:
mkdir public
2. Add a CSS File: Inside the public
folder, create a file named styles.css
:
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
color: #333;
padding: 20px;
}
h1 {
color: #5a5a5a;
}
3. Add a JavaScript File: In the same public
folder, create a file named scripts.js
:
document.addEventListener('DOMContentLoaded', function() {
console.log('JavaScript is working!');
});
4. Serve Static Files: To serve these static files, modify your server.js
file to use Express’s static
middleware:
app.use(express.static(path.join(__dirname, 'public')));
This line tells Express to serve all the files in the public
directory as static files. Now, you can link your CSS and JavaScript files in your HTML files like this:
Welcome to My Node.js Website
Hello, World!
This is an HTML file served by Node.js using Express.
Now, when you run your server, the CSS and JavaScript will be applied to your HTML pages, making your website look and feel more dynamic.
Conclusion
Rendering HTML files in Node.js is a crucial part of web development. Whether you’re building a small personal project or a large-scale web application, understanding how to serve HTML, CSS, and JavaScript files efficiently is key. With Node.js and Express, you have the tools to create powerful and responsive websites with minimal effort.
If you’re interested in learning more about Node.js and web development, there are plenty of resources available. The official Node.js documentation is a great place to start, offering comprehensive guides and tutorials. Additionally, MDN Web Docs provides in-depth articles on web development topics, including Node.js.
By mastering these skills, you’ll be well on your way to becoming a proficient web developer. So go ahead, start experimenting with Node.js, and see what amazing websites you can create!